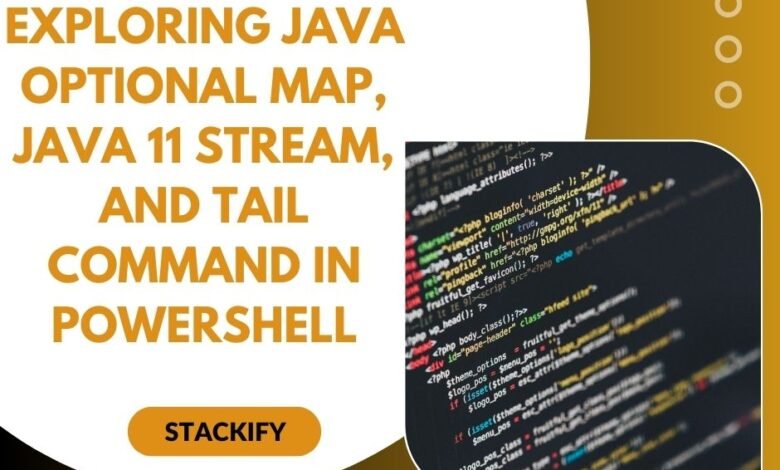
In the ever-evolving world of software development, keeping up with the latest tools and technologies is crucial. Today, we will explore three important concepts that developers frequently encounter: the Optional.map() method in Java, the powerful Stream API introduced in Java 11, and the Tail command in PowerShell. This blog will provide an in-depth understanding of how these tools work and their practical applications, with a focus on making your code cleaner and more efficient.
Understanding Java’s Optional Map Method
Java’s Optional map, introduced in Java 8, is a part of the java.util package. It was designed to handle potential null values and prevent the infamous NullPointerException (NPE). Instead of returning null, methods that may not have a value can return an Optional object. This object can then be safely operated on using a variety of methods, including the Optional.map() method.
What is Optional.map()?
The Optional.map() method is used to transform the value inside the Optional if it is present. The transformation is done using a Function passed as an argument. If the Optional is empty, map() will simply return an empty Optional rather than throwing an exception.
Here’s a basic example:
java
Optional<String> optionalValue = Optional.of(“Stackify”);
Optional<Integer> optionalLength = optionalValue.map(String::length);
optionalLength.ifPresent(System.out::println);
In this example, the Optional.map() method applies the String::length function to the optionalValue. If the Optional contains a string, it maps that value to its length. If the Optional is empty, it simply returns another empty Optional.
Best Practices for Using Optional.map()
- Avoid overusing Optional: While Optional is a great tool, avoid using it as a replacement for all null checks. Use it when there’s a clear need, such as when a method might return no result.
- Use Optional.map() for transformations: When you need to transform a value inside an Optional, always use map() instead of manually checking for null.
- Handle empty Optionals gracefully: Methods like orElse() or orElseGet() can provide a default value if the Optional is empty, ensuring the program doesn’t break.
By using Optional.map(), you can make your code safer and more readable, reducing the risk of NullPointerException and promoting better practices.
Leveraging Java 11 Stream API
The Java 11 Stream API, introduced in Java 8 and improved in later versions, including Java 11, allows developers to process collections of objects in a functional programming style. The API’s focus on declarative programming makes code more readable and concise. Java 11 brought several enhancements to the Stream API that can help you write better code.
Java 11 Stream Enhancements
Java 11 added several enhancements to the Stream API. Two of the most notable additions are:
takeWhile(): This method takes elements from the stream as long as a given predicate is true. Once the predicate is false, it stops processing.
Example:
java
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6);
List<Integer> result = numbers.stream()
.takeWhile(n -> n < 4)
.collect(Collectors.toList());
System.out.println(result); // Output: [1, 2, 3]
dropWhile(): The opposite of takeWhile(), this method drops elements while a given predicate is true, and once it’s false, it processes the remaining elements.
Example:
java
List<Integer> result = numbers.stream()
.dropWhile(n -> n < 4)
.collect(Collectors.toList());
System.out.println(result); // Output: [4, 5, 6]
These methods are especially useful when dealing with ordered streams, where you want to process or exclude elements based on a condition.
Best Practices for Using Java 11 Streams
- Use streams for readability: While streams can make your code more concise, it’s essential to balance brevity with clarity. Avoid long chains of operations that make the code difficult to read.
- Be mindful of performance: Streams can have performance implications when processing large datasets, especially when using operations like filter(), map(), or reduce(). Use parallel streams if necessary to improve performance.
- Favor immutability: Streams promote the use of immutable collections. Whenever possible, avoid mutating the original collections to prevent unexpected behavior.
The Stream API offers a powerful way to work with collections and allows developers to write code that is easier to read and maintain. Java 11’s enhancements like takeWhile() and dropWhile() provide even more flexibility in how streams are processed.
Using the Tail Command in PowerShell
Moving from Java in PowerShell, another powerful tool in a developer’s arsenal is the Tail command. In Unix-like systems, tail is a command-line utility that displays the last few lines of a file. PowerShell, a cross-platform shell, also offers similar functionality, and it is particularly useful when dealing with log files or other files that are frequently updated.
Basic Syntax of Tail in PowerShell
In PowerShell, the tail command can be executed using Get-Content. The -Tail parameter allows you to specify the number of lines you want to display from the end of a file.
Here’s an example:
powershell
Get-Content -Path “C:\Logs\example.log” -Tail 10
This command will show the last 10 lines of the example.log file. If you want to monitor a file in real-time, similar to tail -f in Unix, you can use the -Wait parameter:
powershell
Get-Content -Path “C:\Logs\example.log” -Wait
This command will continue to display new lines as they are added to the file, making it perfect for monitoring logs in real-time.
Best Practices for Using Tail in PowerShell
- Use -Tail for efficient log monitoring: Instead of reading the entire log file, use -Tail to only fetch the last few lines. This can save memory and time, especially with large files.
Combine with filtering commands: You can combine Get-Content with other PowerShell commands like Where-Object to filter log entries based on conditions. For example, to display only error messages, you could use:
powershell
Get-Content -Path “C:\Logs\example.log” -Tail 100 | Where-Object {$_ -like “*error*”}
- Leverage -Wait for real-time log updates: When debugging or monitoring production systems, the -Wait option can be invaluable for staying up-to-date with log activity without having to constantly reopen the file.
Conclusion
In conclusion, whether you’re working with Java’s Optional.map(), Java 11’s enhanced Stream API, or PowerShell’s Tail command, these tools can make your development workflow smoother and more efficient. By following best practices and leveraging the full potential of these features, you can write cleaner, more maintainable code and improve your overall productivity.